こんにちは、fuyutsukiです。
Pythonスクリプトを自動実行した際に、きちんとプログラムが動作しているのかをどうやって確認すれば良いんだ、と疑問に感じたことはありませんか?今回の記事ではそうした悩みを解決しますのでぜひ最後まで読んでください。
また、タスクスケジューラでPythonスクリプトを自動実行する手順の詳細についてはこちらの記事で解説しています。
Pythonスクリプトを自動実行する際のおすすめのログの残し方
pythonでデバッグする際に、print()文を使っている方は多いのではないでしょうか。
そこで、logをテキストファイル形式で出力することをおすすめします。
logをテキストファイルで保存する
こちらのようにpythonコードを記載することでlogをテキスト形式で保存することができます。
ログファイルが重たくなりすぎることを防ぐために、一週間毎にログファイルをリセットするようにしています。
# ログファイルの設定
log_file = "program_log.txt"
def check_log_reset(log_file_name):
"""ログファイルがリセットされるべきかどうかを判断する"""
# ファイルの最終更新日を取得
if os.path.exists(log_file_name):
last_modified = datetime.fromtimestamp(os.path.getmtime(log_file_name))
now = datetime.now()
# ここでリセットの条件を設定 (例: 一週間毎)
if now - last_modified > timedelta(weeks=1):
# 古いログファイルをリネームしてアーカイブ(オプション)
os.rename(log_file_name, f"{log_file_name}_{last_modified.strftime('%Y%m%d')}")
# 新しいログファイルを作成
open(log_file_name, 'w').close()
def log_message(message):
"""ログメッセージをファイルに記録する関数"""
check_log_reset(log_file) # ログファイルのリセットチェックを追加
with open(log_file, "a") as file:
timestamp = datetime.now().strftime("%Y%m%d %H:%M:%S")
file.write(f"{timestamp} - {message}\n")
print(message)
def log_error(message, e):
"""エラーメッセージと例外の詳細をログに記録する関数"""
error_message = f"{message}: {e}\n{traceback.format_exc()}"
log_message(error_message)
log_message('処理を実行しました')
上記のpythonコードを実行すると、”時間”と”処理を実行しました”という単語がlogファイルのテキストファイルに保存されます。
スクリーンショットを撮る
webページを自動で操作するような場合ですと、処理毎にスクリーンショットを撮ることもおすすめです。
GoogleChromeでWebDriverを使用する際に、スクリーンショットを撮る例を示すpythonコードがこちらです。
# screenshotフォルダが存在しない場合は作成
folder_name_screeenshot = 'screenshot'
screeenshot_directory = os.path.join(current_directory, folder_name_screeenshot)
if not os.path.exists(screeenshot_directory):
os.makedirs(screeenshot_directory)
def screenshot_filepath(filename):
screenshot_directory_filepath = os.path.join(current_directory, screeenshot_directory)
# スクリーンショットのファイル名(パスを含む)
screenshot_file_path = os.path.join(screenshot_directory_filepath, filename+".png")
print(screenshot_file_path)
time.sleep(1)
return screenshot_file_path
def safe_screenshot(driver, filename):
try:
# アラートが存在するかをチェック
alert = driver.switch_to.alert
alert_text = alert.text
print(f"Alert found: {alert_text}")
# アラートがあれば、それを解除
alert.accept()
print("Alert has been accepted.")
except NoAlertPresentException:
# アラートが存在しない場合は何もしない
print("No alert present.")
# アラートの処理後にスクリーンショットを取る
screenshot_path = screenshot_filepath(filename)
driver.get_screenshot_as_file(screenshot_path)
# Chromeオプションを設定
chrome_options = Options()
chrome_options.add_argument("--headless") # ヘッドレスモードを有効化
chrome_options.add_argument("--window-size=1920,1080") # ウィンドウサイズを指定
chrome_options.add_argument("log-level=3")
# WebDriverのセットアップ
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()), options=chrome_options)
safe_screenshot(driver, "example")
上記のpythonコードを実行するとexample.pngという名前のスクリーンショットが保存されます。
まとめ:Pythonスクリプトを自動実行するならテキストファイルでログを残そう
今回はPythonスクリプトを自動実行した際に、プログラムがきちんと動作していることを確認するため、ログを残すおすすめの方法について解説しました。
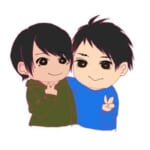
単純ですが、テキストファイルに”時間”と”処理内容”を保存していくのがおすすめです。
私のブログではPythonに関する記事を多く執筆しているため、ぜひまた記事を読みに来てください!